What is a variable?
This is an introduction to PowerShell and so this article will only discuss very basic variable types and information. If you want a more detailed discussion you can find very good information on the MS Docs How to Use PowerShell pages.
Before we start on variable types, I need to make sure you know what we mean by the term “variable”. As an adjective the dictionary definition of that word is “capable of being changed; alterable;” as a noun, in computing terms, it has this definition “a quantity capable of assuming any set of values” – what we are dealing with then is a “thing” that holds a “value” where that value can “change”.
In computing terms that “thing” is normally an area in memory. Computer memory isn’t something humans can easily access so we give the storage space a name and the computer remembers where in memory that name refers to and can read and write to it.
The “Value” is just some data that we choose to store in that “thing”, in our context it could be a piece of text, a number, a date and time or something more complex.
Do you use Excel? Have you ever stored a value in a cell and later referenced that cell by its row and column name such as A1 or B2? If so then you have used a kind of variable.
Naming Variables
In PowerShell we identify a variable by adding the dollar sign to the beginning of its name, good practice suggests that variable names should be easy to understand, consider these two examples:
$name $n
You can’t see what is held in a variable when reading or writing the PowerShell itself, which of these two would more easily identify the data that it holds? Which one would give you a better idea of its contents? If you said $name
then we agree and I’d say you get this concept.
Some other things to note about variable names:
- PowerShell is not case sensitive so
$name
,$NAME
and$Name
are all interchangeable. - You may wish to have longer variable names on occasion, the convention in this case is to use Camel case. Camel case capitalises the first letter of each word to make reading it easier. Consider:
$userprincipalname
and$UserPrincipalName
– which is the easier to read? For most I would venture it is the latter written in camel case. - Spaces are not generally allowed in variable names, they can be used in certain circumstances but should be avoided in your early PowerShell days. If you really need to separate words, convention would use the underscore in a manner similar to these examples,
$First_Name
,$Family_Name
. The general consensus though is that variable names in PowerShell should not contain special characters and I would suggest you follow that convention as you start out.
Assigning Values
Now you know how to pick a good name, we need to assign the value. The most common way to assign a value to a variable is to use the equals sign (=), I did this in the previous post on WMI and CIM cmdlets
$session = New-CIMSession -ComputerName Child-Dc1 -credential film\administrator
You can be more direct
$Name = "Tom"
There are a plethora of examples
$Number = 1 $Service = "wuauserv" $FileName = "C:\Temp\PowerShellDemo.txt" $Circumference = 2 * 3.14 * $Radius
As you can see we can assign different types of data, numbers, strings, calculated objects and Objects from the output of a PowerShell command, let’s look at some of these variable types.
Variable Types
The common variable types used by early PowerShell adopters are most likely
- String (Text enclosed in apostrophes that can include special characters)
- Int (An Integer is a whole number or a number with no decimal places)
- Double (A number that can include decimal places)
- DateTime (A PowerShell object that holds date and time)
- Bool (A simple true or false value)
This is not a ultimate list, there are others, you can have arrays (lists) and hash tables (key-value pairs), objects from the output of PowerShell cmdlets and you can even create your own custom PowerShell Objects, but these are the most common types to start and the principle is the same for all. Once you grasp the principle with these simple types you will easily grow to using others.
Declaring Variables
Some programming languages require that you declare a variable before you can use it and as part of that declaration you must define the type of data that variable will hold. This is not the case with PowerShell. It is possible to define the type of data a variable can hold manually by adding the type in square brackets as part of the declaration or assignment, but it is not compulsory.
[Int]$Number [String]$Name [Bool]$State [Int]$Number = 10 [Bool]$State = $true [String]$Name = "Tom"
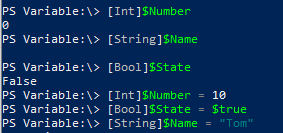
The ability to just “use” variable without having to declare and define them, is because PowerShell can do this for you “behind the scenes”. Doing so, requires it to make an educated guess at the type you need based on the data that you store in it. If you assign the value 1 it will make the variable type Int, if you store the value “1” it will make the variable type string. Be careful, this may catch you out, especially as you start to assign values through the pipeline.
One Last Thing
If you are not assigning values immediately, it is good practice to empty the variable. It may also be necessary, once you start scripting, to empty a variable before its next use. You can do this by assigning the value $null
. This ensures that you are starting with a clean, empty variable and know that any value(s) it contains have been assigned by you, or at least the code you have written.
[Int]$Number = $null [String]$Name = $null
In the next post we will look at how we can use the variables that we create.